The algorithm is the soul of a program and software. As a good programmer, only a comprehensive mastery of some basic algorithms will be handy in the process of designing programs and writing code. This article is the second in a series of nearly 100 C language algorithms, including the classic Fibonacci sequence, simple calculator, palindrome check, prime check and other algorithms. Maybe they can come in handy in your graduation design or interview.
1, calculate the Fibonacci sequence
The Fibonacci series, also known as the Fibonacci sequence, is also known as the golden section, which refers to such a sequence: 1, 1, 2, 3, 5, 8, 13, 21.
The code for the C language implementation is as follows:
/* Displaying Fibonacci sequence up to nth term where n is entered by user. */ #include
Int main() { int count, n, t1=0, t2=1, display=0; printf("Enter number of terms: "); scanf("%d",&n); printf("Fibonacci Series: % d+%d+", t1, t2); /* Displaying first two terms */ count=2; /* count=2 because first two terms are already displayed. */ while (count Result output: Enter number of terms: 10 Fibonacci Series: 0+1+1+2+3+5+8+13+21+34+ You can also use the following source code:
/* Displaying Fibonacci series up to certain number entered by user. */ #include Int main() { int t1=0, t2=1, display=0, num; printf("Enter an integer: "); scanf("%d",&num); printf("Fibonacci Series: %d+%d+ ", t1, t2); /* Displaying first two terms */ display=t1+t2; while(display Result output: Enter an integer: 200 Fibonacci Series: 0+1+1+2+3+5+8+13+21+34+55+89+144+ 2. Back to text check Source code:
/* C program to check whether a number is palindrome or not */ #include Int main() { int n, reverse=0, rem,temp; printf("Enter an integer: "); scanf("%d", &n); temp=n; while(temp!=0) { rem= Temp%10; reverse=reverse*10+rem; temp/=10; } /* Checking if number entered by user and it's reverse number is equal. */ if(reverse==n) printf("%d is a palindrome .",n); else printf("%d is not a palindrome.",n); return 0; } Result output:
Enter an integer: 12321 12321 is a palindrome. 3. Prime check Note: 1 is neither a prime nor a composite.
Source code:
/* C program to check whether a number is prime or not. */ #include Int main() { int n, i, flag=0; printf("Enter a positive integer: "); scanf("%d",&n); for(i=2;i<=n/2;++ i) { if(n%i==0) { flag=1; break; } } if (flag==0) printf("%d is a prime number.",n); else printf("%d is Not a prime number.",n); return 0; } Result output:
Enter a positive integer: 29 29 is a prime number. 4. Print pyramids and triangles Use * to create a triangle
* * * * * * * * * * * * * * * Source code:
#include Int main() { int i,j,rows; printf("Enter the number of rows: "); scanf("%d",&rows); for(i=1;i<=rows;++i) { For(j=1;j<=i;++j) { printf("* "); } printf(""); } return 0; } Use the digital print half pyramid as shown below.
1 1 2 1 2 3 1 2 3 4 1 2 3 4 5 Source code:
#include Int main() { int i,j,rows; printf("Enter the number of rows: "); scanf("%d",&rows); for(i=1;i<=rows;++i) { For(j=1;j<=i;++j) { printf("%d ",j); } printf(""); } return 0; } Print semi-pyramids with *
* * * * * * * * * * * * * * * Source code:
#include Int main() { int i,j,rows; printf("Enter the number of rows: "); scanf("%d",&rows); for(i=rows;i>=1;--i) { For(j=1;j<=i;++j) { printf("* "); } printf(""); } return 0; } Print pyramid with *
* * * * * * * * * * * * * * * * * * * * * * * * * Source code:
#include Int main() { int i,space,rows,k=0; printf("Enter the number of rows: "); scanf("%d",&rows); for(i=1;i<=rows;+ +i) { for(space=1;space<=rows-i;++space) { printf(" "); } while(k!=2*i-1) { printf("* "); ++ k; } k=0; printf(""); } return 0; } Print inverted pyramid with *
* * * * * * * * * * * * * * * * * * * * * * * * * Source code:
#include Int main() { int rows,i,j,space; printf("Enter number of rows: "); scanf("%d",&rows); for(i=rows;i>=1;--i) { for(space=0;space Source code: /* Source code to create a simple calculator for addition, subtraction, multiplication and division using switch...case statement in C programming. */ # include Int main() { char o; float num1,num2; printf("Enter operator either + or - or * or divide : "); scanf("%c",&o); printf("Enter two operands: "); Scanf("%f%f",&num1,&num2); switch(o) { case '+': printf("%.1f + %.1f = %.1f",num1, num2, num1+num2); break ; case '-': printf("%.1f - %.1f = %.1f",num1, num2, num1-num2); break; case '*': printf("%.1f * %.1f = % .1f",num1, num2, num1*num2); break; case '/': printf("%.1f / %.1f = %.1f",num1, num2, num1/num2); break; default: / * If operator is other than +, -, * or /, error message is shown */ printf("Error! operator is not correct"); break; } return 0; } Result output:
Enter operator either + or - or * or divide : - Enter two operands: 3.4 8.4 3.4 - 8.4 = -5.0 6. Check if a number can be expressed as the sum of two prime numbers Source code:
#include Int prime(int n); int main() { int n, i, flag=0; printf("Enter a positive integer: "); scanf("%d",&n); for(i=2; i< =n/2; ++i) { if (prime(i)!=0) { if ( prime(ni)!=0) { printf("%d = %d + %d", n, i, ni ); flag=1; } } } if (flag==0) printf("%d can't be expressed as sum of two prime numbers.",n); return 0; } int prime(int n) /* Function to check prime number */ { int i, flag=1; for(i=2; i<=n/2; ++i) if(n%i==0) flag=0; return flag; Result output:
Enter a positive integer: 34 34 = 3 + 31 34 = 5 + 29 34 = 11 + 23 34 = 17 + 17 7. Reverse the string recursively Source code:
/* Example to reverse a sentence entered by user without using strings. */ #include Void Reverse(); int main() { printf("Enter a sentence: "); Reverse(); return 0; } void Reverse() { char c; scanf("%c",&c); if( c ! = '') { Reverse(); printf("%c",c); } } Result output:
Enter a sentence: margorp emosewa awesome program 8. Realize the conversion between binary and decimal /* C programming source code to convert either binary to decimal or decimal to binary according to data entered by user. */ #include #include Int binary_decimal(int n); int decimal_binary(int n); int main() { int n; char c; printf("Instructions:"); printf("1. Enter alphabet 'd' to convert binary to decimal." Printf("2. Enter alphabet 'b' to convert decimal to binary."); scanf("%c",&c); if (c =='d' || c == 'D') { printf ("Enter a binary number: "); scanf("%d", &n); printf("%d in binary = %d in decimal", n, binary_decimal(n)); } if (c =='b ' || c == 'B') { printf("Enter a decimal number: "); scanf("%d", &n); printf("%d in decimal = %d in binary", n, decimal_binary( n)); } return 0; } int decimal_binary(int n) /* Function to convert decimal to binary.*/ { int rem, i=1, binary=0; while (n!=0) { rem=n% 2; n/=2; binary+=rem*i; i*=10; } return binary; } int binary_decimal(int n) /* Function to convert binary to decimal.*/ { int decimal=0, i=0, Rem; while (n!=0) { rem = n%10; n/=10; decimal += rem*pow(2,i); ++i; } return decimal; } Result output:
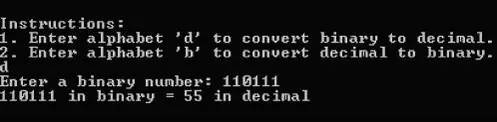
9, using multidimensional arrays to achieve the addition of two matrices Source code:
#include Int main(){ int r,c,a[100][100],b[100][100],sum[100][100],i,j; printf("Enter number of rows (between 1 and 100 ): "); scanf("%d",&r); printf("Enter number of columns (between 1 and 100): "); scanf("%d",&c); printf("Enter elements of 1st matrix :"); /* Storing elements of first matrix entered by user. */ for(i=0;i Result output: 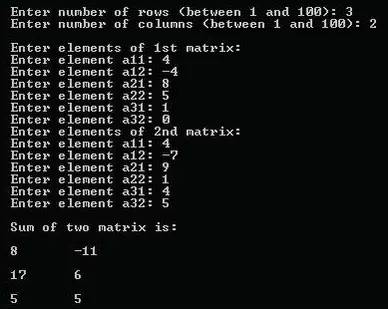
10. Matrix transposition Source code:
#include Int main() { int a[10][10], trans[10][10], r, c, i, j; printf("Enter rows and column of matrix: "); scanf("%d %d ", &r, &c); /* Storing element of matrix entered by user in array a[][]. */ printf("Enter elements of matrix:"); for(i=0; i From: Code Farm Network Link: http://
Lana Vape E-liquids
LANA Vape E-liquids is so convenient, portable, and small volume, you can choose the flavors you like, then add into your lana vape.
We are China leading manufacturer and supplier of Disposable Vapes puff bars, lana vape e-liquids 30ml,lana vape e-liquids energy,
lana vape e liquids e-cig,lana vape e-liquids for pods, and e-cigarette kit, and we specialize in disposable vapes, e-cigarette vape pens,
e-cigarette kits, etc.
lana vape e-liquids 30ml,lana vape e-liquids energy,lana vape e liquids e-cig,lana vape e-liquids for pods,lana vape e-liquids salt nic
Ningbo Autrends International Trade Co.,Ltd. , https://www.mosvapor.com